.velato-js
10 Nov 2014Velato is an esoteric programming language based on principles of music theory. I wasn't able to use the .NET compiler to try out the language so I made velato.js, a transpiler which takes in Velato code and spits out JavaScript. In the process I learned a bit about transpilers, language design and the obsession of an ancient mathematician..
.velato primer
Velato uses terms native to music theory which I didn't understand. Even the description of the language required me to open up multiple wikipedia tabs and basic music theory tutorials. I found that in order to print the letter H in Velato it took two thousand years of Western music history.
It is hard to jump straight into Velato unless you are both a talented musician and a computer programmer. I am not a talented musician so I went about learning some basic music theory which I used with Velato. I am going to try explaining those basics then show how to print the letter "H" in Velato. Yes, only the letter "H". I understand "Hello World" is more customary but I am lazy.
For me, learning Velato began with Pythagoras possibly walking past a blacksmith. He came up with a bunch of theories about string length and perfect integers which were adopted by western music terminology. There is a wealth of interesting and slightly occult theories from Pythagoras on this subject but for Velato I was only interested in the idea of a Chromatic Scale[2].
The chromatic scale is the basis of how Velato recognizes commands. A Velato program begins by the programmer (me) choosing what is called a "root note". This note is used to measure the distance in semitones to other notes in the command.
For instance, if I choose C as my root note then 9 semitones from C is the note A and 7 semitones from C is the note G.
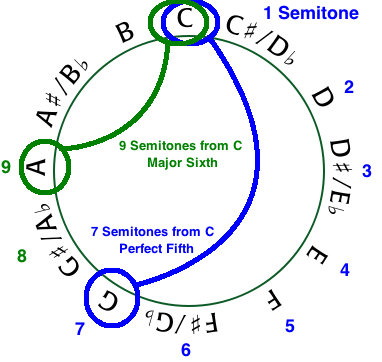
The semitone distances map to what are called intervals. The interval names I used can be found in the velato.js source code where every number is associated with the number of semitones and the corresponding interval name.
At this point the list of Velato commands makes more sense to me. Each command begins with a "root note" and then expects a pattern of semitone distances to match a command. In this case I start with the root note of "C" and follow it up with "A G" to create a print command.
The rest of the syntax falls into place with the expressions not caring about Major or Minor and literals being mapped to a sequence of intervals. At this point some of the more complex examples in the tests make a bit more sense although they hurt my brain.
At this point I can code in Velato but the difficult part about the language isn't writing code. The difficulty for me is trying to make the code I write sound good. It is a unique experience to consider if my conditional statement should use a different variable name to make it more rhythmic.
.try programming velato
The editor below takes input as VexTab notation and executes the notes using velato.js, the output is shown below the editor.
.parsed velato
.velato output
.how velato-js works
Overview:
- Raw Velato code is input, e.g. "C A G"
- String is tokenized to individual characters ["C", "A", "G"]
- Characters are matched against either a command or an expression
- The matched command or expression is buffered as the next match
- The match is converted to an AST element
- The AST is sent to escodegen
- escodegen returns a string containing executable javascript
The process starts by using a Lexer to split up the input string. The Lexer is responsible for turning strings of notes into tokens. Each token is a set of notes which makes up a command or expression. A token will record the current root note, the matched note and the location of the note in the string.
When the Lexer returns a statement (Velato command or expression) the parser attempts to find a parselet which is responsible for a statement of that name. If a statement is found then the statement tries to parse the next statement, this is where the recursion begins matching the rest of the language. As long as each token matches to a statement then the process continues until the end of the source file.
The statements being matched are returned as Abstract Syntax Tree nodes (AST nodes). I used the Spider Monkey Parser API AST node syntax as the representation for each statement. At this point the entire AST can be viewed to check for operator precedence. I used the escodegen demo to compare the AST generated with the one generated using plain JavaScript.
escodegen takes as input a Spider Monkey Parser API AST and returns as output a string of executable JavaScript. As long as I can generate an AST in the format which Spider Monkey Parser expects then I can use escodegen to make executable JavaScript.
The technique to transpile Velato code is one which can be used to create any sort of transpiler to JavaScript. A Pratt parser, lexer and escodegen make it possible to quickly bootstrap a complex transpiler which is able to be used in the browser.
.libraries used
- Spider Monker Parser API
- escodegen
- escodegen demo
- Pratt Parser
- Pratt Parser in Coffeescript
- Improved escodegen
.some useful links
These aren't all on transpilers, some are libraries I used in order to make things work or I found cool.